A guide to use python with pepper the robot
Joshua Inglis
Choregraphe is an excelent tool for rapid prototyping of pepper applications. However with python, you can do a lot more with pepper with less overhead and with quicker runtimes.
Python basics
When coding, you'll use functions. A lot. This is because you can executed many lines of code and reuse code with a single line. A function has this form
def my_function():
some code
Lets unpack this part. The def keyword indicates you want to define a function. my_function is the name of the function, you can name your function whatever you want, as long as it doesn't share the same name as a pre existing function. () is where you put your parameters. They will be delt with later. The Some code will be executed after the function name is called. For exaple if I have the following code
def say_hello_world():
print("hello world")
say_hello_world()
The first two lines define the function say_hello_world, and indicates that print("hello world") will be excecuted when say_hello_world is executed. The 4th line calls the say_hello_world function which executes everything under the say_hell_world function.
The python box
First we create an empty python box by slecteing python box in the 'create a box' menu of the right click menu. Double click on the new box to access the coding area. The box will already have four functions; __init__, onload, onUnload, onInput_onStart, onInput_onStop. Onload is called when the applications starts and ie, when the play button is pressed and onInput_onstart is called when the block is activated. The other functions will be covered later.
Our First Applications
Our first application will make pepper say hello world. self.ALProxy("ALTextToSpeach") is the class that allows pepper to speak. We will asign self.tts to self.ALProxy("ALTextToSpeach") to make it quicker to access text to speach and make it easier for people to read. We do this by typing following under onload(), indented by one.
def onLoad():
self.tts = self.ALProxy("ALTextToSpeach")
and in onInput_onStart write
self.tts.say('Hello world')Press play and the robot should say 'hello world'. For more on text to speech click here
Logging
A very important part of any programming is logging, which alows for messages to be sent to to a log menu to identiy the you code while running to identify bugs. There are four different types of logs, debug, info and error, the function of each are self explanitory. To make a write the following:
self.logger.info("message")Where message is the text to be outputted to the log menu.
Using boxes
While this guide will go through how to use python and creating own own boxes, it's still handy to use the pre-built boxes in choregraphe to make our lives easiser. First a box input my be created by right clicking on the box and creating an input called my_input.
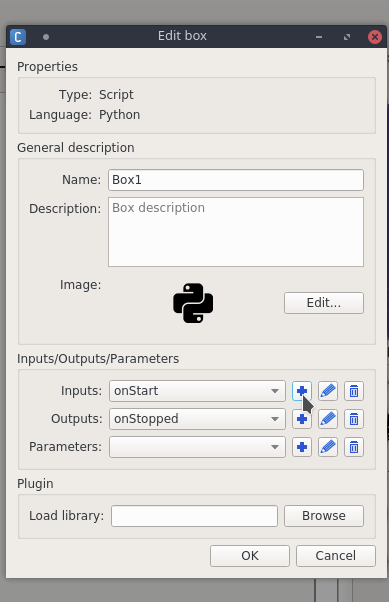
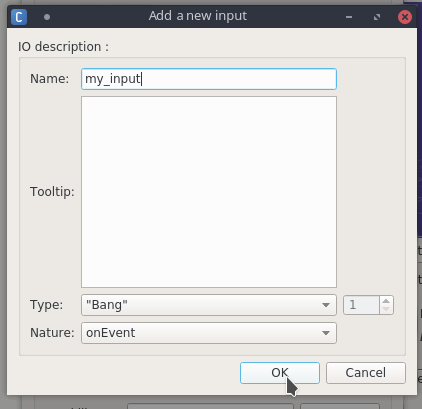
In to accept box inputs we use the following code
self.my_input(): #some codeThe first line of the above code must be in line with the other def statements. This is a function, we've seen then before as they prepopulated the python box. When a function is called the code underneath the function definition line (def my_function():) is executed. For more on functions click here. Now when a box is activated with a line, this function will be called.
To execute an output we must make an output on the box.
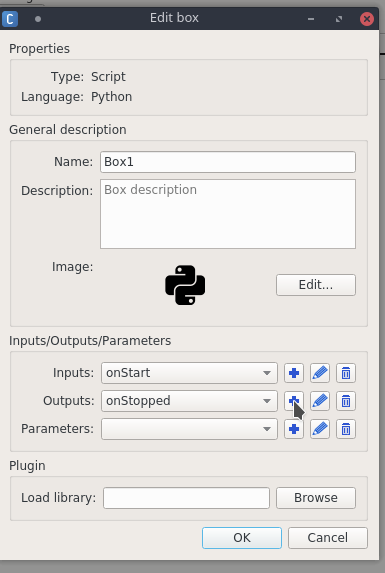
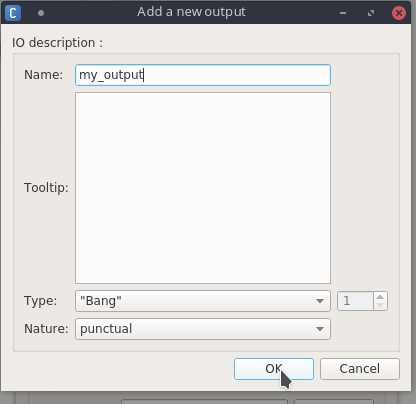
Practice activity
Now you are to create your own application. Without using a say block and only using one python create an application that says 'you touched the top of my head' when the top of head is tapped, 'you touched the middle of my head' when the middle of the head is touched, and 'you touched the back of my head' when the back of its head is touched. Also when the back of it's head is touched, it's eyes twinckle. You will need the the tacktile had block and the twincle block.